Notice
Recent Posts
Recent Comments
Link
Hello It's good to be back ^_^
[ECC-프론트엔드 2팀] 16주차 스터디 본문
교재: 소플의 처음 만난 리액트 11 ~ 13
공부한 페이지: pp. 318 ~ 386
실습한 내용: https://github.com/HongYeonLee/ECC-Frontend-Study
GitHub - HongYeonLee/ECC-Frontend-Study: ECC 49기 FW 프론트엔드 2팀 스터디 내용을 기록하는 레포지토리입
ECC 49기 FW 프론트엔드 2팀 스터디 내용을 기록하는 레포지토리입니다. Contribute to HongYeonLee/ECC-Frontend-Study development by creating an account on GitHub.
github.com
목차
11 폼
- 11.1 폼이란 무엇인가?
- 11.2 제어 컴포넌트
- 11.3 textarea 태그
- 11.4 select 태그
- 11.5 File input 태그
- 11.6 여러 개의 입력 다루기
- 11.7 Input Null Value
- 11.8 사용자 정보 입력받기
12 State 끌어올리기
- 12. 1 Shared State
- 12.2 하위 컴포넌트에서 State 공유하기
- 12.3 섭씨온도와 화씨온도 표시하기
13 합성 VS. 상속
- 13.1 합성에 대해 알아보기
- 13.2 상속에 대해 알아보기
- 13.3 Card 컴포넌트 만들기
실습1 - 이름 입력받기
코드
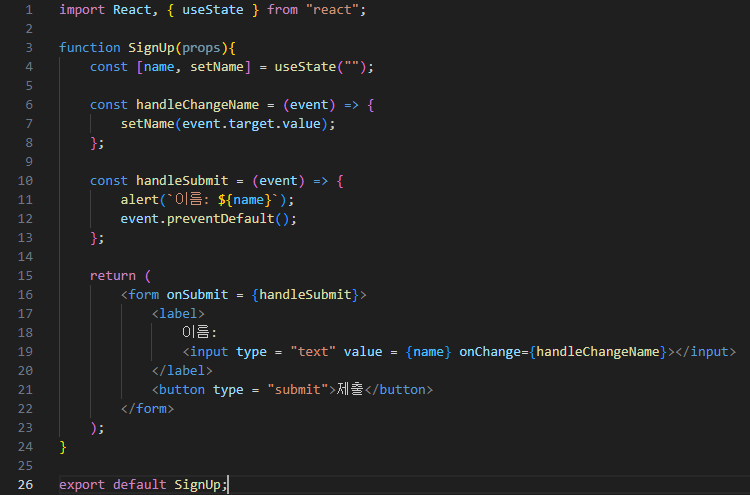
import React, { useState } from "react";
function SignUp(props){
const [name, setName] = useState("");
const handleChangeName = (event) => {
setName(event.target.value);
};
const handleSubmit = (event) => {
alert(`이름: ${name}`);
event.preventDefault();
};
return (
<form onSubmit = {handleSubmit}>
<label>
이름:
<input type = "text" value = {name} onChange={handleChangeName}></input>
</label>
<button type = "submit">제출</button>
</form>
);
}
export default SignUp;
실행화면
실습2 - 성별 입력받기
코드
import React, { useState } from "react";
function SignUp(props){
const [name, setName] = useState("");
const [gender, setGender] = useState("여자");
const handleChangeName = (event) => {
setName(event.target.value);
};
const handleChangeGender = (event) => {
setGender(event.target.value);
}
const handleSubmit = (event) => {
alert(`이름: ${name}, 성별: ${gender}`);
event.preventDefault();
};
return (
<form onSubmit = {handleSubmit}>
<label>
이름:
<input type = "text" value = {name} onChange={handleChangeName}></input>
</label>
<br></br>
<label>
성별:
<select value={gender} onChange={handleChangeGender}>
<option value="여자">여자</option>
<option value="남자">남자</option>
</select>
</label>
<button type = "submit">제출</button>
</form>
);
}
export default SignUp;
실행화면
실습 - 섭씨온도와 화씨온도 표시하기
코드
const scaleNames = {
c: "섭씨",
f: "화씨",
};
function TemperatureInput(props){
const handleChange = (event) => {
props.onTemperatureChange(event.target.value);
};
return (
<fieldset>
<legend>
온도를 입력해 주세요 (단위: {scaleNames[props.scale]}):
</legend>
<input value={props.temperature} onChange={handleChange}/>
</fieldset>
);
}
export default TemperatureInput;
import React, { useState }from "react";
import TemperatureInput from "./TemperatureInput";
function BoilingVerdict(props){
if(props.celsius >= 100){
return <p>물이 끓습니다</p>;
}
return <p>물이 끓지 않습니다.</p>;
}
function toCelsius(fahrenheit) {
return ((fahrenheit - 32) * 5) / 9;
}
function toFahrenheit(celsius){
return (celsius * 9) / 5 + 32;
}
function tryConvert(temperature, convert){
const input = parseFloat(temperature);
if(Number.isNaN(input)){
return "";
}
const output = convert(input);
const rounded = Math.round(output * 1000) / 1000;
return rounded.toString();
}
function Calculator(props){
const [temperature, setTemperature] = useState("");
const [scale, setScale] = useState("c");
const handleCelsiusChange = (temperature) => {
setTemperature(temperature);
setScale("c");
};
const handleFahrenheitChange = (temperature) => {
setTemperature(temperature);
setScale("f");
};
const celsius =
scale === "f" ? tryConvert(temperature, toCelsius) : temperature;
const fahrenheit =
scale === "c" ? tryConvert(temperature, toFahrenheit) : temperature;
return (
<div>
<TemperatureInput
scale="c"
temperature={celsius}
onTemperatureChange={handleCelsiusChange}
/>
<TemperatureInput
scale="f"
temperature={fahrenheit}
onTemperatureChange={handleFahrenheitChange}
/>
<BoilingVerdict celsius={parseFloat(celsius)}></BoilingVerdict>
</div>
);
}
export default Calculator;
실행화면
실습 - Card 컴포넌트 만들기
코드
function Card(props){
const { title, backgroundColor, children } = props;
return (
<div style = {{
margin: 8,
padding: 8,
borderRadius: 8,
boxShadow: "0px 0px 4px grey",
backgroundColor: backgroundColor || "white",
}}
>
{title && <h1>{title}</h1>}
{children}
</div>
);
}
export default Card;
import Card from "./Card";
function ProfileCard(props){
return(
<Card title = "Hongyeon Lee" backgroundColor = "#4ea04e">
<p>안녕하세요, 이홍연입니다.</p>
<p>저는 리액트를 사용해서 개발하고 있습니다.</p>
</Card>
);
}
export default ProfileCard;
실행내용
'Study > 프론트엔드' 카테고리의 다른 글
[ECC-프론트엔드 2팀] 17주차 스터디 (1) | 2025.01.25 |
---|---|
[ECC-프론트엔드 2팀] 17주차 스터디 (3) | 2025.01.16 |
[ECC-프론트엔드 2팀] 15주차 스터디 (1) | 2025.01.04 |
[ECC-프론트엔드 2팀] 14주차 스터디 (2) | 2024.12.28 |
[ECC-프론트엔드 2팀] 13주차 스터디 (1) | 2024.12.21 |